Debugging reigns supreme as the ultimate problem-solving skill. It’s the art of unraveling perplexing errors, the science of pinpointing flaws in code, and the cornerstone of software development. This article is your compass through the debugging wilderness, offering insights into proven strategies, essential tools, and the mindset needed to conquer bugs with confidence. From reproducing glitches to implementing fixes, we’ll navigate the debugging journey together, empowering you to troubleshoot like a seasoned pro and emerge victorious in the battle against bugs.
Common phrases developers say when debugging:
Why isn’t it working?
It was working just moments ago!
OMG, it was a semicolon causing the issue this whole time!
It’s working on my machine.
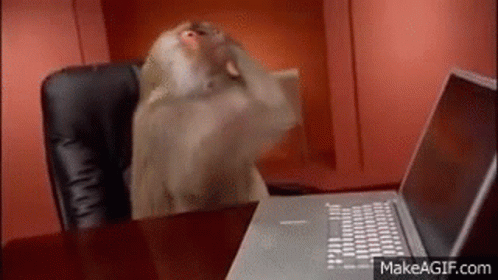
Understanding Debugging
Debugging is the process of finding and fixing problems in software code. It’s like being a detective, where developers investigate why their code isn’t working as expected and make corrections to ensure it runs smoothly.
Significance in Software Development
Debugging is crucial because it:
- Improves Code Quality: By resolving issues early, developers create cleaner and more efficient code.
- Enhances User Experience: Debugging ensures applications work correctly, providing users with a better experience.
- Saves Time and Resources: Fixing bugs promptly prevents costly delays and rework in the development process.
- Maintains Reputation: Effective debugging helps uphold a company’s reputation for delivering high-quality software.
Common Causes of Bugs
- Syntax Errors: Mistakes in code structure or language rules, like misspelled commands or missing punctuation.
- Logic Errors: Flaws in the code’s logic or algorithms that lead to incorrect outcomes, often challenging to detect.
- Runtime Errors: Issues that occur during program execution, such as unexpected conditions or exceptions, causing the program to crash or behave unexpectedly.
Understanding these causes helps developers tackle bugs efficiently, ensuring their software works as intended.
The Debugging Process
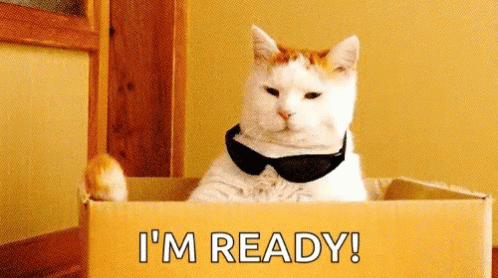
Reproduce the Bug
Recreating the bug consistently is the first step in fixing it. Here’s why it matters and how to do it easily:
Understanding the Problem
Seeing the bug happen again and again helps us understand what’s causing it. It’s like finding the root of the issue.
Simplifying the Test
Make a simple setup that only focuses on the problem. Strip away anything unnecessary to see the bug clearly.
Cutting Out Distractions
Ignore things that aren’t related to the bug. By focusing only on the parts of the code involved, we can fix it faster.
Taking Notes
Write down the steps to make the bug happen. This helps us remember exactly how to trigger it and share the info with others.
By making the bug happen consistently and creating a simple test case, we set ourselves up to understand and fix it faster.
Diagnose the Problem
Identifying bugs can be tricky, but a systematic approach makes it easier:
Code Review
- Carefully check your code for obvious mistakes or confusing logic.
- Team up with others for a fresh set of eyes during code reviews.
Step-by-Step Execution
- Use your coding environment to execute the code one step at a time.
- Watch how the program behaves at each step to catch any unexpected issues.
Print Statements
- Insert print statements in key places to show variable values and program flow.
- Analyse the printed output to find where things might be going wrong.
Logging
- Integrate logging to record runtime information, errors, and warnings.
- Categorise messages by severity to focus on debugging-relevant information.
Debugging Tools
- Explore built-in debugging tools to set breakpoints and inspect the program’s state.
- Pause execution to check variable values and understand the code flow.
Unit Testing and Test Cases
- Create tests to check individual parts of your code.
- Automate tests to catch regressions or unexpected behavior.
Code Profiling
- Use profiling tools to identify performance issues like slow code or high memory use.
- Optimise your code based on the tool’s insights.
Error Messages and Stack Traces
- Pay attention to error messages and stack traces.
- Understand the sequence of function calls leading to the error to pinpoint the problem.
By combining these methods, you can break down complex issues, catch mistakes early, and fix bugs more efficiently.
Fixing the Bug
Once you know what’s causing the problem, it’s time to make things right. Here’s how:
Fixing It Up
- Think carefully about how to solve the problem. Keep it simple and easy to understand.
- Write clear explanations in your code about why you’re making these changes. It helps others (and your future self!) understand what’s going on.
Testing, Testing, Testing
- Before saying “problem solved,” test your fix to make sure it actually works. Try different scenarios to see if the issue is truly gone.
- Use tools to help you test automatically, so you can find any new problems your fix might create.
- Sometimes, you just need to eyeball it – manually test the fix, especially if it involves stuff like how things look on the screen.
Double-Check Everything
- Even after you think you’ve fixed it, check again! Make sure your fix didn’t accidentally break something else.
- Test not just what you fixed, but also other parts of your program to see if they’re still working fine.
Putting It Out There
- Once you’re confident your fix is solid, it’s time to share it with the world. Follow the rules for sharing code in your team or company.
- Keep an eye on things after you’ve made the fix. Just to be sure everything’s running smoothly.
By following these simple steps, you’ll be able to squash those bugs and keep your code running smoothly!
Effective Debugging Strategies
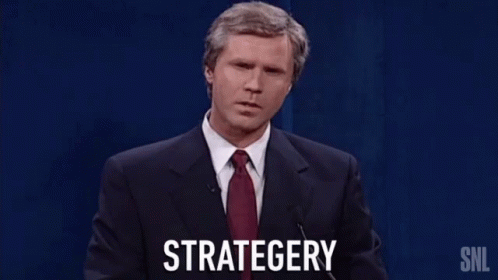
Divide and Conquer
When bugs pop up in code, they can feel overwhelming. But with the divide and conquer strategy, developers can break them down into smaller, easier-to-handle pieces.
Breaking it Down
Instead of tackling the whole problem at once, break it into smaller parts. This makes it easier to understand and fix.
Finding the Culprit
Once you’ve divided the problem, focus on one part at a time. This helps narrow down where the bug might be hiding.
Spotting the Bug
By isolating specific pieces of code, you can zoom in on the bug’s location. This makes it quicker to find and fix.
Imagine a slow website. Instead of fixing everything at once, focus on parts like database or webpage loading. This helps pinpoint the exact issue and fix it faster. Divide and conquer is a smart way to deal with bugs. It simplifies the problem, making it easier to solve step by step.
Rubber Duck Debugging
Rubber Duck Debugging is a trick where you talk through your code problem with, well, a rubber duck (or any inanimate object). It sounds silly, but explaining the issue out loud helps you spot mistakes and find solutions.
Here’s why it works:
- Clear Thinking: Talking about the problem forces you to break it down step by step, making it easier to understand.
- Thoroughness: Explaining it out loud makes you consider every detail, uncovering hidden issues.
- New Insights: Speaking engages your brain differently, often leading to fresh ideas and solutions.
- Self-Sufficiency: Trying to solve it yourself first boosts your skills and confidence.
So, grab your duck and start chatting. You might be amazed at how helpful it can be!
Debugging Tools
Debugging tools are like superpowers for programmers, making it easier to find and fix mistakes in their code. By using tools like IDE debuggers, profilers, and memory analysers, developers can build better software faster and with fewer headaches.
IDE Debuggers
- Features in your coding software (like breakpoints and step-by-step execution) help you pause your code and see what’s going on inside.
- Useful for finding mistakes in how your code runs and checking values of variables.
Profilers
- Tools that help find which parts of your code are slow or use a lot of computer memory.
- Great for making your programs run faster and smoother by finding and fixing performance issues.
Memory Analysers
- Tools to check if your code is using too much computer memory or not releasing it properly.
- Useful for preventing memory-related problems like crashes or slowdowns.
Developing a Debugging Mindset
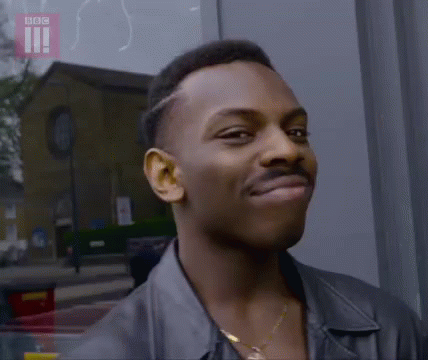
Patience and Persistence
In programming, bugs are like puzzles — sometimes tricky, but always solvable. When you hit a snag, it’s crucial to keep two things in mind: patience and persistence.
Patience
Bugs can be sneaky, hiding in the nooks and crannies of your code. It might take a few tries to find them, but don’t get frustrated. Take your time and work through the problem step by step. Remember, patience pays off in the end.
Persistence
Debugging is a bit like detective work. Even when things seem tough, keep at it. Try different approaches, ask for help if you need it, but don’t give up. With each attempt, you’re getting closer to cracking the case.
Patience and persistence are essential traits for any programmer. So, next time you’re faced with a tricky bug, remember to take a deep breath, stay calm, and keep at it. You’ve got this!
Continuous Learning
Bugs happen in coding, but they’re not just problems – they’re chances to learn. When you encounter a bug:
- Understand the Why: Instead of just fixing the surface issue, figure out why it happened. This helps you avoid similar problems in the future.
- Try Different Things: Experiment with different solutions to see what works. Even if you don’t fix it right away, you’ll learn something new in the process.
- Write It Down: Keep notes on what you tried and what worked. This helps you remember for next time and lets you improve your skills over time.
Exploring New Ways to Debug
Keep learning about new debugging tools and tricks:
- Stay Updated: Read up on the latest debugging methods through blogs, forums, and workshops.
- Try New Tools: Experiment with different debugging tools to see which ones work best for you.
- Join the Community: Talk to other developers to learn from their experiences and share your own.
- Keep Getting Better: Treat debugging like a skill that gets better with practice. Keep trying new things and learning from your mistakes.
By seeing bugs as learning opportunities and staying open to new ways of debugging, you’ll become a more confident and effective coder.
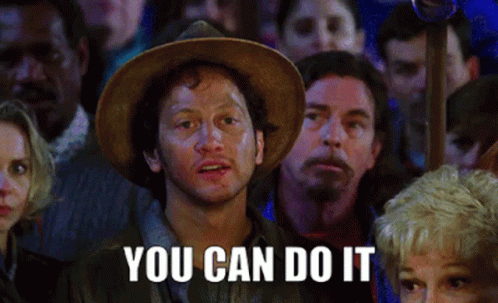
In this article, we’ve explored the art of debugging in programming, emphasising its importance and offering strategies for success. From understanding the nature of bugs to employing techniques like dividing and conquering complex problems, we’ve discussed how debugging is not just about fixing errors but also about developing patience and persistence. By approaching debugging with confidence and a proactive mindset, developers can sharpen their skills and tackle any coding challenge that arises, knowing that with practice and experience, they’ll become adept at unraveling even the most stubborn bugs. Happy debugging!